Helper Functions and Program Logic
This page serves as a guide to the helper functions used within the programs generated by Verbotics Weld, and the logic which these programs follow.
Program Logic
Verbotics Weld utilises multiple essential helper functions that make up the core logic path of a verbotic’s welding program. This logic path is illustrated below:
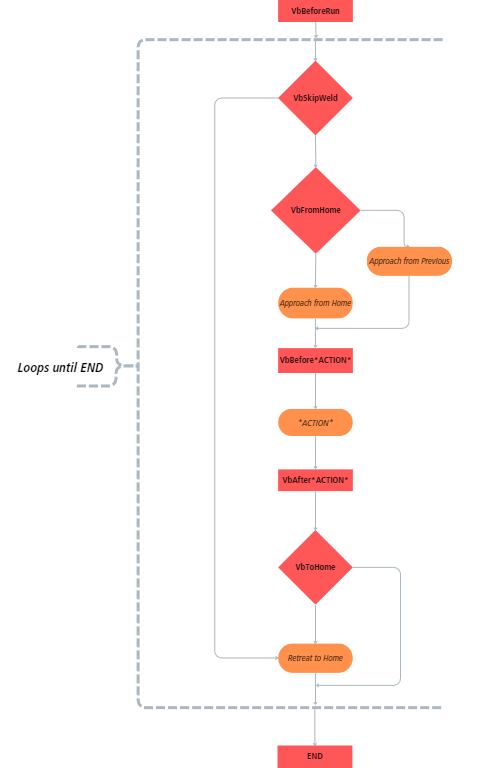
Program Logic Flowchart
Note: ACTION represents either a welding or sensing motion. E.g. (VbAfterWeld)
Following the diagram above, the program heavily relies on the 3 essential boolean helper-functions to direct your programs path. These being VbSkipWeld, VbFromHome and VbToHome:
VbSkipWeld: Allows the program to determine if the proceeding weld should be skipped or completed.
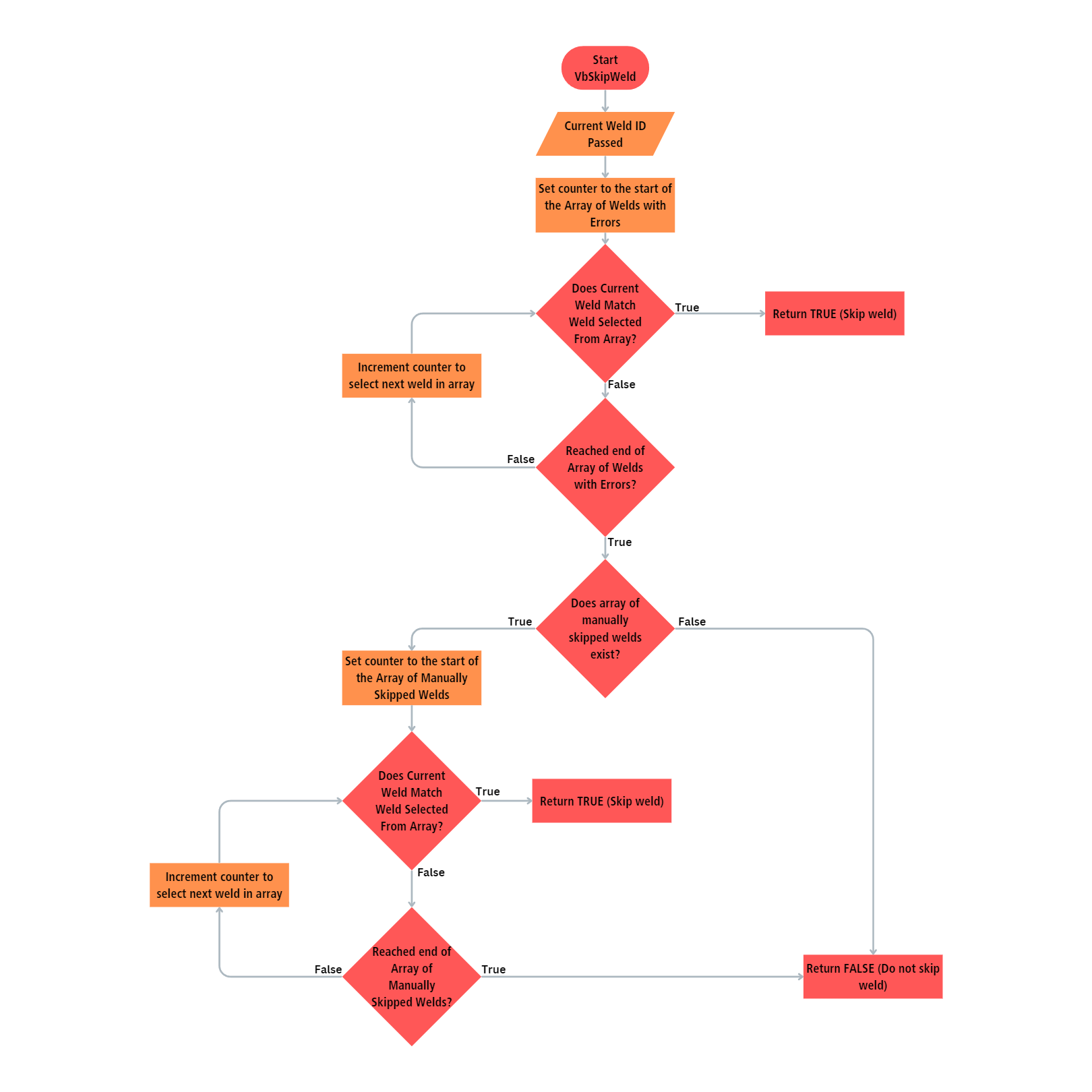
Flowchart for the Skip Welds Helper Function
VbFromHome: Informs the program whether the tool will be approaching the next action (e.g. sense, weld) from a home position, or from the previous position where it finished its last action.
VbToHome: Allows the program determine if the robot can retain its current position, or if it needs to return to its set home position.
Note
A weld should only ever be skipped when the program has commanded the robot back to the home position. Skipping a weld from any other location will result in potential collisions.
In addition to these 3 decision-based helper functions, there are also several helper functions which complete specific processes at different points of the program:
VbBeforeRun: Executes any additional code within the function before the start of the program and initialises robot-specific variables.
VbAfterRun: Executes any additional code within the function after the end of the program.
VbBeforeTouchSense: Executes any additional code within the function before starting a touch sense motion and sets the searchError flag to false.
VbAfterTouchSense: Executes any additional code within the function after completing a touch sense motion and handles search errors if found.
VbBeforeWeld: Executes any code within the function before the start of a welding motion.
VbAfterWeld: Executes any code within the function after the end of a welding motion.
Examples
The following examples present several scenarios that may occur in your programs. The provided pseudocode on the left shows the steps the program follows, while on the right a diagram illustrates the logic path as the program advances.
Manual Weld Skip
A client wants to manually skip welds 1 and 2 in their program. To do this, an array containing these 2 welds is included at the start of the program, and every time skip welds is called, the current weld ID is checked against this array.
This exemplifies how you can include an array consisting of any welds you wish to skip and how the logic of this situation should work.
|
![]() Program Logic Example |
Perform Weld Range
Skip on Sense Error
For this example, the program detects an error during the sensing stage of weld 2 and as a result returns home before skipping weld 2 and advancing to the sensing of weld 3.
This exemplifies how when an error is detected, the program will record the current WeldID that raised the error in an array. This array is used to determine when the program needs to return home and skip the relevant weld.
|
![]() Program Logic Example |
Complete List of Helper Functions
Below is a list of the helper functions common across all robots. The naming below is based off the ABB robot naming convention, however for the the specific helper function names for your robot, see the relevant system integrator page for your robot.
Helper Function Name |
Definition |
Use |
---|---|---|
VbBeforeRun |
Executes any additional code within the function before the start of the program and initialises robot-specific variables |
Add the code specific to your robot here, and any additional code you wish to execute prior to program start |
VbAfterRun |
Executes any additional code within the function after the end of the program |
Include any additional code you wish to run after the welding program has completed here. |
VbBeforeTouchSense |
Executes any additional code within the function before starting a touch sense motion and sets the searchError flag to false. |
Called prior to starting a touch sense motion, include any code relating to touch sense preparation or specific adjustments here. |
VbAfterTouchSense |
Executes any additional code within the function after completing a touch sense motion and handles search errors if found. |
Called after a touch sense motion has been completed, in addition to the error detection and handling, can also run user-added code prior to error handling. |
VbBeforeWeld |
Executes any code within the function before the start of a welding motion |
Called prior to a welding motion, add any specific welding operation adjustments or code here. |
VbAfterWeld |
Executes any code within the function after the end of a welding motion |
Called after a welding motion, include any post-weld code in this procedure. |
VbSkipWeld |
Retrieves the weld data of the weld being skipped, if an error is detected for that weld then that weld is skipped. Otherwise, the weld is undertaken. Robot MUST be returned to home position before calling this procedure |
Called at the start of every loop to determine if the next weld is to be skipped. Should only ever be called after VbToHome to ensure the robot is returned to its home position before skipping a weld |
VbToHome |
Returns the robot to the home position if a search error occurs, else the robot remains in its current position. |
Called to determine if any errors exist for the current weld, if an error is identified, returns the robot to the home position. |
VbCutWire |
Executes additional code related to cutting the robot wire, robot MUST be returned to home position before calling this procedure. |
Called only after the robot has returned to the home position and executes your robot’s specific wire cutting code that is to be included in this procedure. |
WbCleanTorch |
Executes additional code related to cleaning the torch, robot MUST be returned to home position before calling this procedure. |
Called only after the robot has returned to the home position and executes your robot’s specific torch cleaning code that is to be included in this procedure. |
VbModifyToolData |
Modifies the tool data using the extra code added to this procedure. |
Optional: Include any tool data modifications in this function to return an updated tool with the new data. |
VbModifyWObjData |
Modifies the wobjdata using the extra code added to this procedure. |
Optional: Include any desired adjustments to the work object data in this function to return an updated wobj to the program. |
VbSearchL |
Conducts the robots search motion using touch sense in order to return an updated offset for increased accuracy. |
Called whenever a sensing motion is required, allows program to retrieve an updated offset to be used when welding. Uses touch-sense to complete this. |
VbLaserSearchL |
Conducts the robots search motion using laser sense in order to return an updated offset for increased accuracy. |
Called whenever a sensing motion is required, allows program to retrieve an updated offset to be used when welding. Uses laser-sense to complete this. |